Before we start
- What will I learn?
Some things will be familiar to you (OOP, C-like programming language, etc) But a number of things will likely be very new to some of you …
Swift Programming Language
Functional Programming and “structured” Programming
“Reactive” User-Interface Development Paradigm (Including MVVM)
Object-Oriented Databases
- Prerequisite
Experience writing code (Preferably you know more than one language)
But it’s not a requirement.
Let’s get start
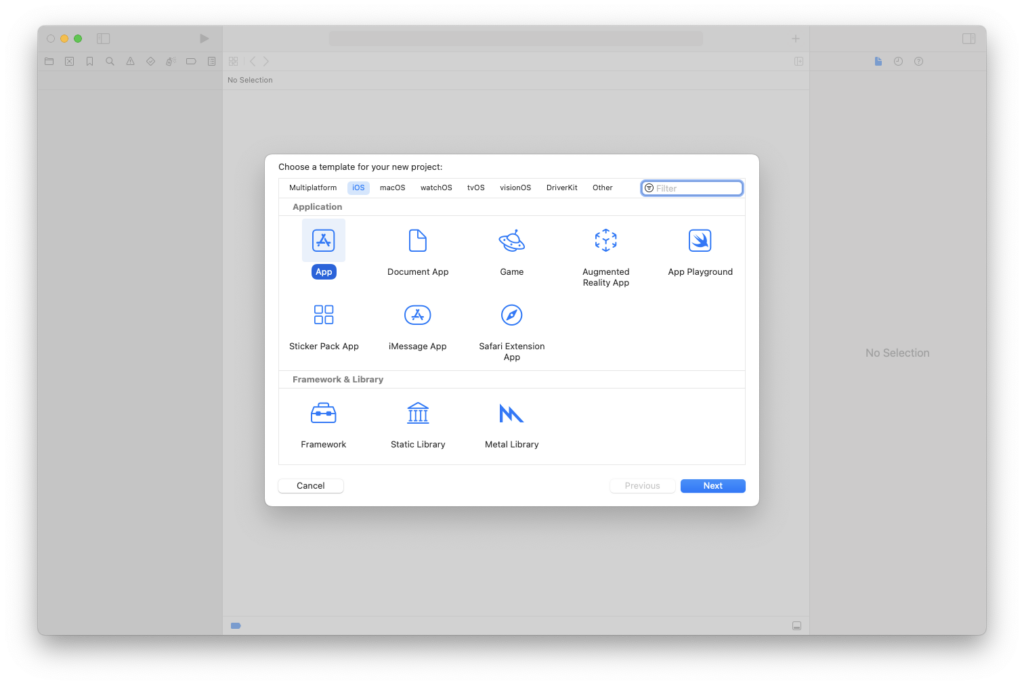
Run Xcode and select App.
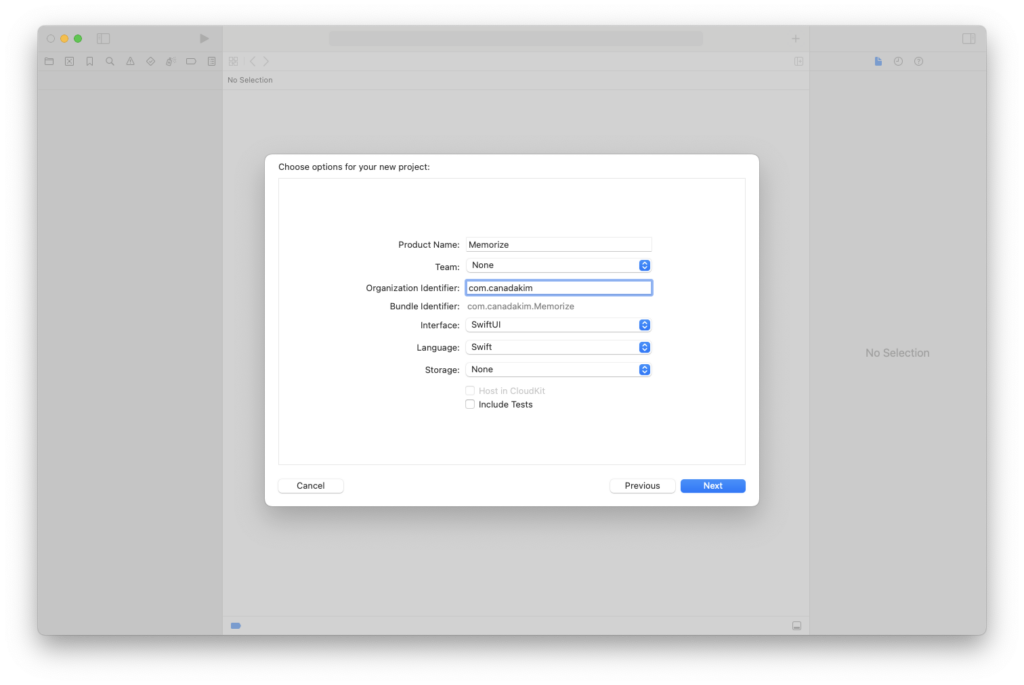
Input your project name in Product Name.
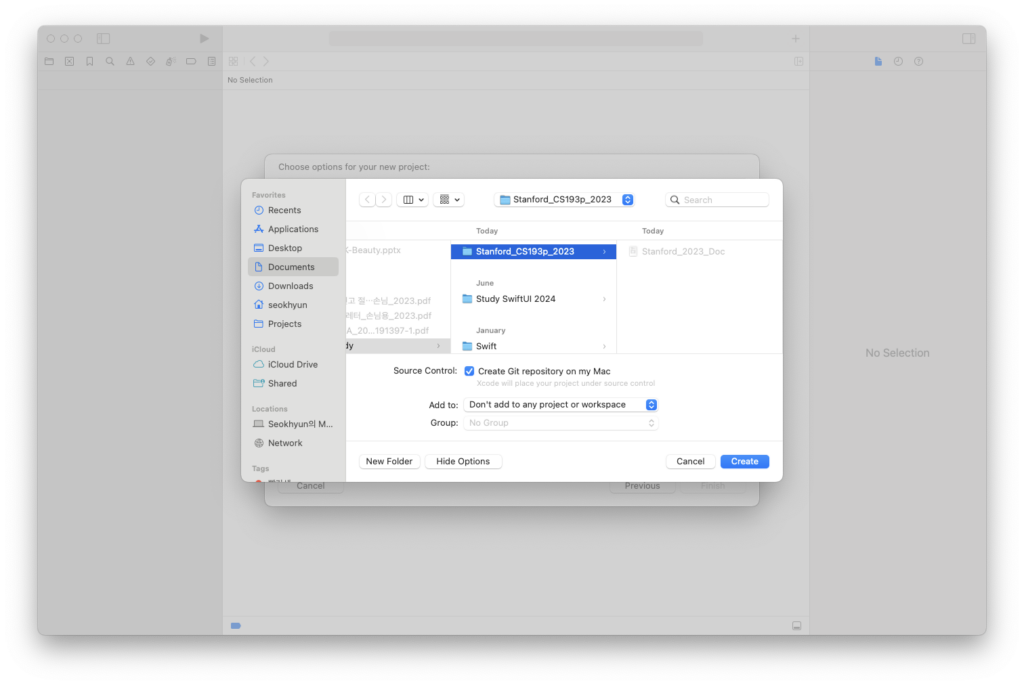
Choose your project folder and create.
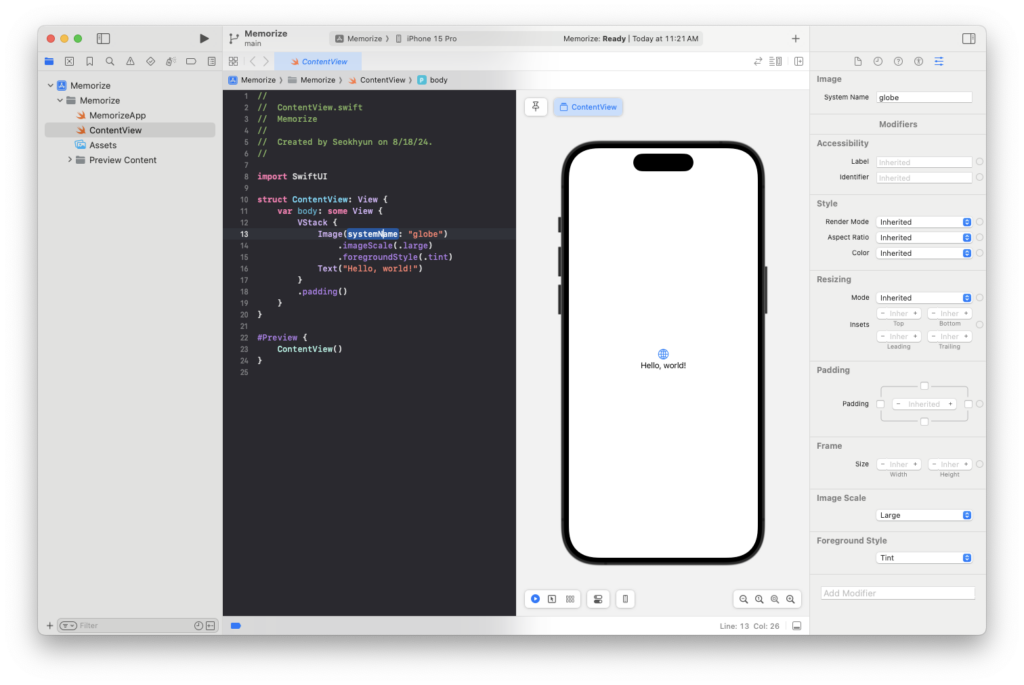
You can see the screen something like that.
What is mean “ContentView”
import SwiftUI
특정 모듈을 사용한다고 선언한다. 즉 “import CoreData” 라고 정의하면 데이타베이스를 사용할 수 있다. 만일 로직을 구현 할 때 UI View를 사용하지 않으면 굳이 import 할 필요가 없다.
struct ContentView: View { ... }
struct : 매우 중요, Swiftui 모든 부분에서 쓰임. 단 class의 OOP와 혼동되지 말자. Swift 문법에서 자세히 다룰 예정입니다.
ContentView : struct 이름, 이름을 수정할 수 있다.
View : “ContentsView behaves like a ..” 함수형 프로그램의 특징, ContentView 는 뷰 처럼 동작(기능)합니다. View에 관한 모든 동작이나 설정을 할 수 있다.
var s: String 변수 s는 구조체이다. 하지만 View는 프로토콜이다. 프로토콜 또한 Swift 문법에서 다룰 예정입니다.
var body: some View { … }
Computed property, 어디에선가 이 { } 안을 요청할 때 마다 계산된다. 값만 계산할 수 있어 읽기 전용 변수와 동일. 즉 var body 읽기 전용 변수라 이름을 수정 할 수 없음. 그리고 다수의 View를 반환한다.
some View : View 처럼 작동하는 무든 구조체의 모임? (레고블럭)
VStack { } or VStack(content: { ... })
이 역시 View 처럼 작동하는 구조체, 여러 다른 뷰를 포함 할 수 있음.
VStack(alignment: .leading, spacing: 20) { … } : argment을 넣어 앞쪽으로 정렬 할 수 있음.
VStack( ) { … } : 빈 ( ) 라고 명시해도 컴파일러가 알아서 해 줌.
{ } 는 function이다. 함수형 프로그래밍에서 함수는 항상 다른 곳에 인수로 전달 됩니다. 함수형 프로그램의 기본
TupleView인 이미지와 택스트라는 두가지 항목을 반환하는 함수이다. 즉 레고를 담는 bag이라고 생각하면 된다.
이 목록을 TupleView로 바꾸는 것은 ViewBuilder 이라고 합니다. 즉 ViewBuilder는 2개이상의 뷰의 집합이다.
Image(systemName: “globe”)
Creating instances of structs for image. image 구조체 또한 뷰의 일종이다.
Named parameters 구조체를 생성하기 위해 다양한 인수 조합을 가질 수 있음.
Text(“hello Kim!”)
Parameter defaults Text는 파라미터의 기본값을 가짐.
.imageScale(.large), .foregroundcolor(.orange)
View modifier 라고 하며 이미지에 대한 속성을 설정 할 수 있다. 이를 아래와 같이 수정 해 보자.
.imageScale(.small), .foregroundcolor(.green)
Text(“Hello Kim!”).padding()
Text 주변을 패딩으로 채움. 패팅에 대해서는 차차 알아가 보자.
var body: some View {
VStack {
Image(systemName: "globe")
Text("Hello, Kim!").padding()
}
.font(.largeTitle)
.imageScale(.large)
View Modifier scope라 하여 VStack의 속성에 font를 largeTitle로 이미지를 크게 설정하였다. 이는 모든 VStack에 있는 뷰에 해당되며 유연성을 가질 수 있다.